In this article, we show how to run a Python script on a server.
- Python Simulator
- Run Python
- Www.programiz.com › Python-programming › Online-compilerOnline Python Compiler (Interpreter) - Programiz
- Console Output
- Html Test Runner Python
- Online Python IDE is a web-based tool powered by ACE code editor. This tool can be used to learn, build, run, test your python script. You can open the script from your local and continue to build using this IDE.
- Run-js currently only supports running functions that accept JSON-serializable input. In other words, you can only call a function that accepts numbers, string, arrays, and simple objects. You can’t pass functions or sets as a parameter.
- If you encounter the any problem running python HTML.py classes under apache on windows you can type this line in the script after 'import HTML': html '. This may sound noobish, but took a while to figure out.
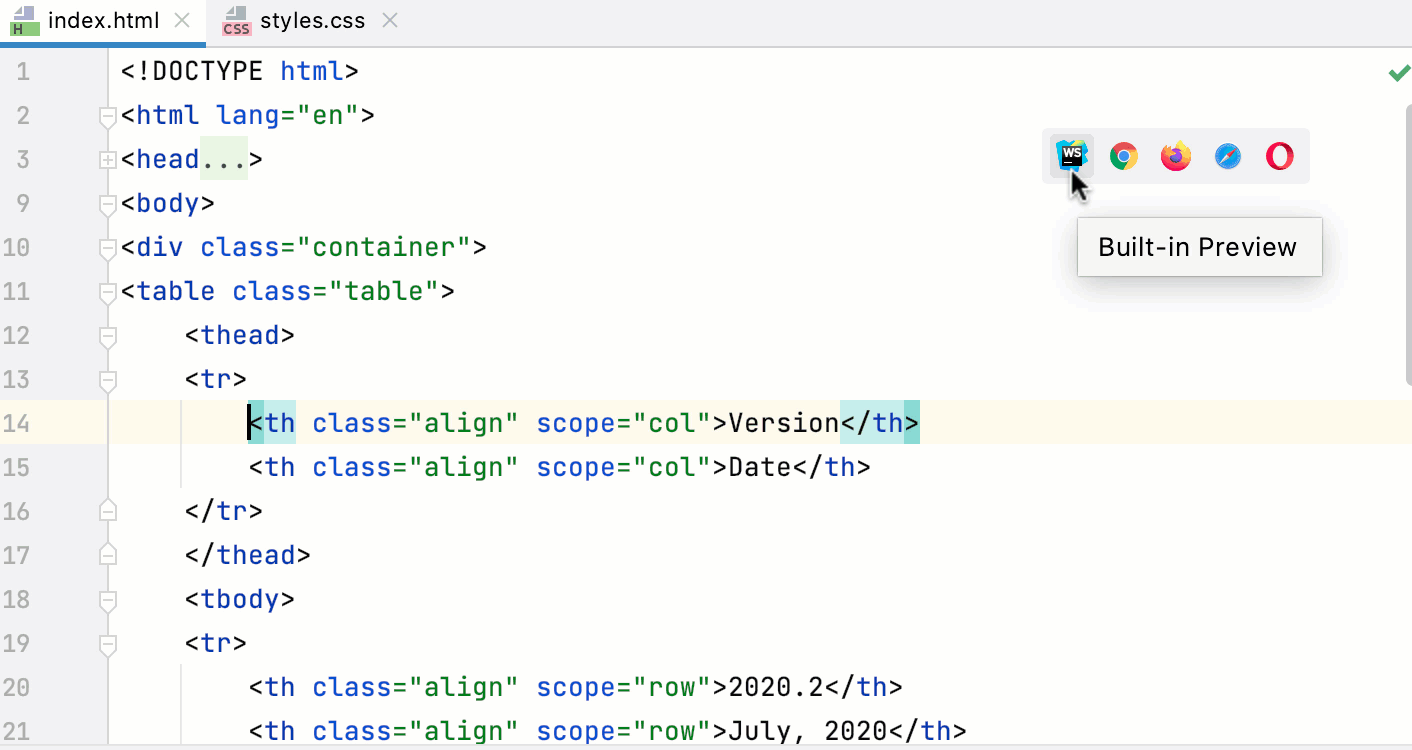
HTMLTestRunner is an extension to the Python standard library's unittest module. It generates easy to use HTML test reports. See a sample report here. HTMLTestRunner is released under a BSD style license. 14 comments Download. HTMLTestRunner.py (0.8.2) testHTMLTestRunner.py test and demo of HTMLTestRunner.py. Return to my software.
So we're going to run this Python script on a server hosted by godaddy.
Many web hosting companies install a Python interpreter on the server. This way, your website that you hostfrom this company can run Python scripts.
In order to run a Python script, you just need to do a few tweaks in a few places.
So let's create a Python file. Let's call it test.py.
In the following code below, we show the contents of this test.py file.
So the first thing we do is specify the location of the Python interpreter on the web server.
Usually the Python interpreter is located in the bin directory.
The bin directory is the directory in linux that contains the ready-to-run programs (also known as the executables). It is here that most likely will contain the Python interpreter for a web server. This directory also contains most of the basic Unix commands such as ls and cop. Most of the programs in the /bin directory are in binary formta, having been created by a C compiler.
So this is a very important line of code. We must specify where the Python interpreter on the server is located, or else the Python code will not work.
After this, we just have to specify that the file is an HTML file.
This allows HTML tags to be executed, since the file is an HTML file. Without this, none of the HTML commands will be executed.
Next we simply print out the statement in h1 tags, This is the home page
Since we want to show that the website can actually run Python code, we run a for loop that iterates 5 timesthrough the loop.
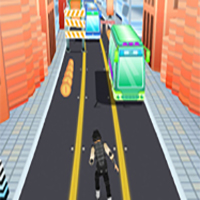
From this for loop, we print out each number of the line. We then print out anything.
Again, this is just to show that Python code can be run on a server.
One last thing, most web hosting companies, including godaddy, do not yet have support for Python 3 (I don't know why). So you have to use Python 2 syntax in order to run Python code. You can see that above we have Python 2 syntax in regardto the print statement. To print in Python 2, we don't use parentheses. But in Python 3, we do. So in the above code, weleave out the parentheses and simply put the quotation marks.
So after you have this Python file (.py file), then you can upload it to any directory on your website.
Once the file is uploaded to the website, then you need to give this file certain permissions.
This is not just a regular static file. Since this file is a Python file and contains Python code, we needto have execute permissions on this file. Remember this is not just a static HTML file. With a scripting language such asPython, it's dynamic. It needs to execute Python code.
So I used the filezilla FTP software in order to upload this Python file to the website.
Once it is uploaded, right click the file and click 'File Permissions...'
This will open up a new window. In this new window, change the Numeric value to 755.
Python Simulator
The numeric value of 755 gives execute permissions to the file, so that Python can execute on it.
Once you have done this, you go go to the web browser and type in the path to the file.
You should now see the Python code which we wrote executed.
This is shown in the output below.
And this is how to run Python code on a web server.
Related Resources
How to Randomly Select From or Shuffle a List in Python
Before you start
Ensure that the following prerequisites are met:
You are working with PyCharm Community or Professional
You have installed Python itself. If you're using macOS or Linux, your computer already has Python installed. You can get Python from python.org.
To get started with PyCharm, let’s write a Python script.
Create a Python project
If you’re on the Welcome screen, click New Project. If you’ve already got any project open, choose File | New Project from the main menu.
Although you can create projects of various types in PyCharm, in this tutorial let's create a simple Pure Python project. This template will create an empty project.
Choose the project location. Click button next to the Location field and specify the directory for your project.
Also, deselect the Create a main.py welcome script checkbox because you will create a new Python file for this tutorial.
Python best practice is to create a virtualenv for each project. In most cases, PyCharm create a new virtual environment automatically and you don't need to configure anything. Still, you can preview and modify the venv options. Expand the Python Interpreter: New Virtualenv Environment node and select a tool used to create a new virtual environment. Let's choose Virtualenv tool, and specify the location of the environment and the base Python interpreter used for the new virtual environment.
When configuring the base interpreter, you need to specify the path to the Python executable. If PyCharm detects no Python on your machine, it provides two options: to download the latest Python versions from python.org or to specify a path to the Python executable (in case of non-standard installation).
Refer to Configure a Python interpreter for more details.
Now click the Create button at the bottom of the New Project dialog.
If you’ve already got a project open, after clicking Create PyCharm will ask you whether to open a new project in the current window or in a new one. Choose Open in current window- this will close the current project, but you'll be able to reopen it later. See the page Open, reopen, and close projects for details.
Create a Python file
In the Project tool window, select the project root (typically, it is the root node in the project tree), right-click it, and select File | New ....
Select the option Python File from the context menu, and then type the new filename.
PyCharm creates a new Python file and opens it for editing.
Edit Python code
Let's start editing the Python file you've just created.
Run Python
Start with declaring a class. Immediately as you start typing, PyCharm suggests how to complete your line:
Choose the keyword
class
and type the class name,Car
.PyCharm informs you about the missing colon, then expected indentation:
Note that PyCharm analyses your code on-the-fly, the results are immediately shown in the inspection indicator in the upper-right corner of the editor. This inspection indication works like a traffic light: when it is green, everything is OK, and you can go on with your code; a yellow light means some minor problems that however will not affect compilation; but when the light is red, it means that you have some serious errors. Click it to preview the details in the Problems tool window.
Let's continue creating the function
__init__
: when you just type the opening brace, PyCharm creates the entire code construct (mandatory parameterself
, closing brace and colon), and provides proper indentation.If you notice any inspection warnings as you're editing your code, click the bulb symbol to preview the list of possible fixes and recommended actions:
Let's copy and paste the entire code sample. Click the copy button in the upper-right corner of the code block here in the help page, then paste it into the PyCharm editor replacing the content of the Car.py file:
This application is intended for Python 3
class Car: def __init__(self, speed=0): self.speed = speed self.odometer = 0 self.time = 0 def say_state(self): print('I'm going {} kph!'.format(self.speed)) def accelerate(self): self.speed += 5 def brake(self): if self.speed < 5: self.speed = 0 else: self.speed -= 5 def step(self): self.odometer += self.speed self.time += 1 def average_speed(self): if self.time != 0: return self.odometer / self.time else: pass if __name__ '__main__': my_car = Car() print('I'm a car!') while True: action = input('What should I do? [A]ccelerate, [B]rake, ' 'show [O]dometer, or show average [S]peed?').upper() if action not in 'ABOS' or len(action) != 1: print('I don't know how to do that') continue if action 'A': my_car.accelerate() elif action 'B': my_car.brake() elif action 'O': print('The car has driven {} kilometers'.format(my_car.odometer)) elif action 'S': print('The car's average speed was {} kph'.format(my_car.average_speed())) my_car.step() my_car.say_state()
At this point, you're ready to run your first Python application in PyCharm.
Run your application
Use either of the following ways to run your code:
Right-click the editor and select Run 'Car' from the context menu .
Press Ctrl+Shift+F10.
Since this Python script contains a main function, you can click an icon in the gutter. If you hover your mouse pointer over it, the available commands show up:
If you click this icon, you'll see the popup menu of the available commands. Choose Run 'Car':

PyCharm executes your code in the Run tool window.
Here you can enter the expected values and preview the script output.
Note that PyCharm has created a temporary run/debug configuration for the Car file.
Www.programiz.com › Python-programming › Online-compilerOnline Python Compiler (Interpreter) - Programiz
The run/debug configuration defines the way PyCharm executes your code. You can save it to make it a permanent configuration or modify its parameters. See Run/debug configurations for more details about running Python code.
Summary
Congratulations on completing your first script in PyCharm! Let's repeat what you've done with the help of PyCharm:
Console Output
Created a project.
Created a file in the project.
Created the source code.
Ran this source code.
Html Test Runner Python
In the next step, learn how to debug your programs in PyCharm.